The goal of this session is to understand how hardware is interfaced with software. Starting from the data sheet of the MAX7219 used to drive an LED matrix, we will first write a kind of driver for it, then some functions to address individual pixels on it, and finally a program to play the Game of Live on an 8 by 8 display.
We have an ESP32 board and an 8 by 8 LED matrix driven by a MAX7219. Here is the wiring (which may vary depending on the development board you use), for an ESP32 DevKit V4 board by Espressif:
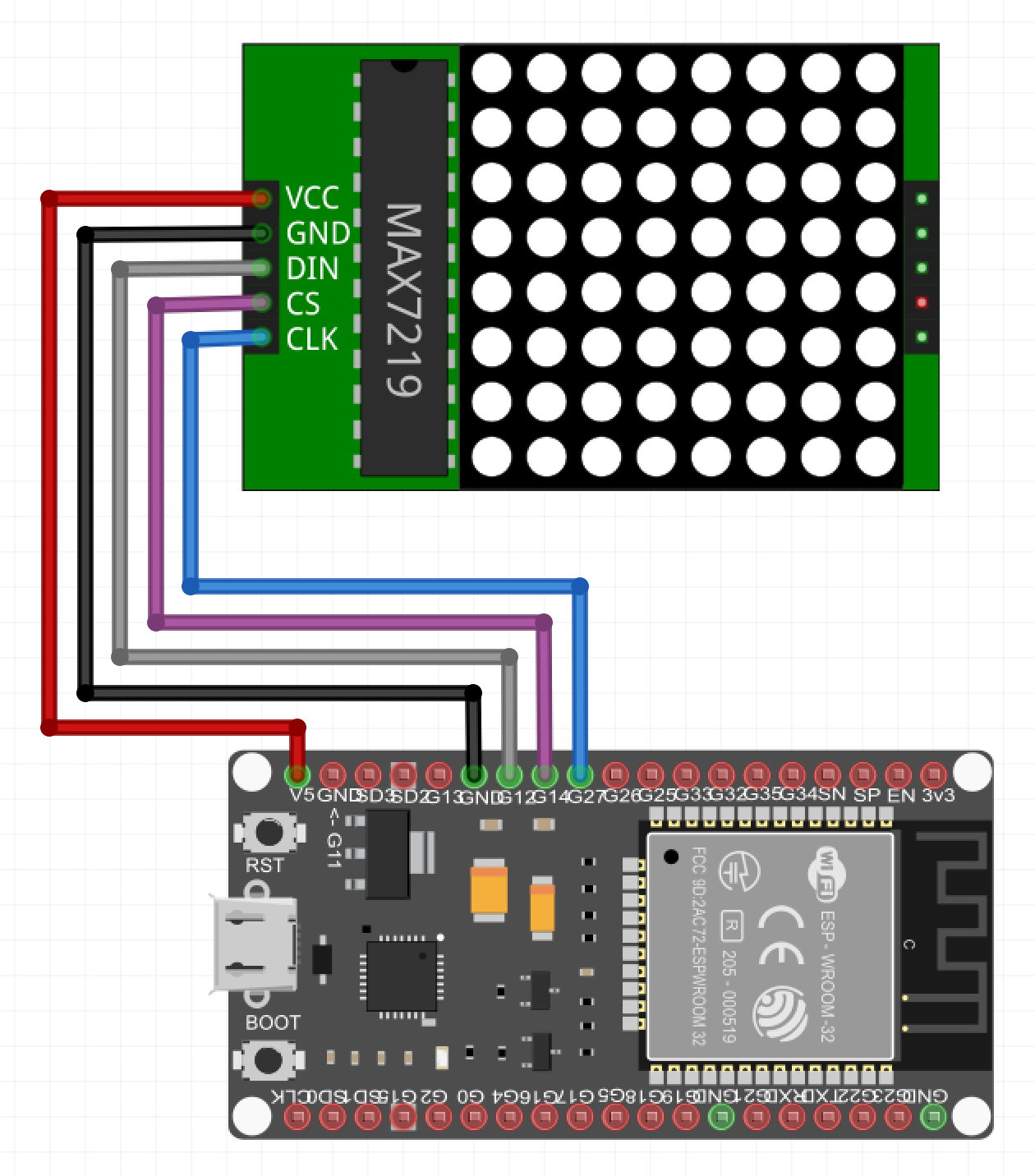
Communication with the MAX7219
The MAX7219 communicates through a synchronous serial link according to the following chronogram:

For instance, to write the data 0x01 into register 0x0F of the MAX7219, which puts it in test mode, we should have the following transmission:
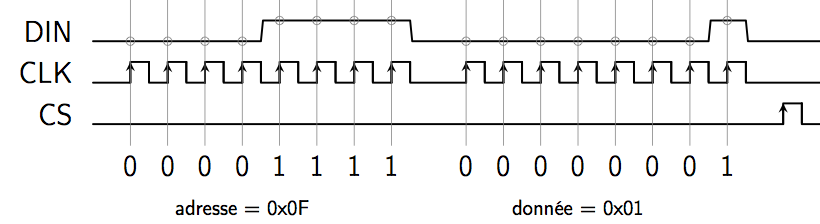
This can be achieved with custom code as follows:
This can also be achieved using the SPI bus interface of the ESP32:
The rest of the practical session works the same as in the original version using the pyboard. You just have to replace calls to pyb.delay(ms)
by calls to time.sleep_ms(ms)
.